.center # Go ## static duck typing ### and more... .meta[Alexander Solovyov, PyGrunn 2012] --- # Go * Announced on November 2009 - Go 1 was released on 28 March 2012 * Ken Thompson, Rob Pike - C, Unix, Plan 9, etc * Conservative, pragmatic --- # Conservative * `{` braces `}` * imperative * static typing * "unhurried" innovativity --- # Pragmatic * fast compilation * garbage collection * convenient type system * concurrency primitives * extensive standard library * UTF-8 --- # Typing system type Printable interface { String() string } func Print(x Printable) { println(x.String()) } --- # Typing system (error) type Page struct { Text string } func main() { page := &Page{"test"} // Type error Print(page) } --- # Typing system (fixed) type Page struct { Text string } func (page *Page) String() string { return page.Text } func main() { page := &Page{"test"} Print(page) } --- # Concurrency func fib(n int) int { // ... return result } func main() { ch := make(chan int) go func() { ch <-fib(5) } println(<- ch) } --- # More! func main() { ch := make(chan int) for i := 1; i <= 10; i++ { go func(n int) { ch <-fib(n) }(i) } for i := 1; i <= 10; i++ { println(<-ch) } } --- # Handling errors func main() { f, err := os.Open("path") if err != nil { log.Fatal(err) } defer f.Close() ... } --- # HTTP daemon, Python .python class Responder(web.RequestHandler): def get(self): name = self.get_argument('id') try: with file(os.path.join(DIR, name)) as f: content = f.read() data = {"content": content} except IOError: data = {"error": "data not found"} self.write(json.dumps(data)) app = web.Application([(r'/', Responder)]) app.listen(8080, 'localhost') ioloop.IOLoop.instance().start() --- # HTTP daemon, Go .small func getContent(name string) map[string]string { data, err := ioutil.ReadFile(filepath.Join(DIR, name)) if err == nil { return map[string]string{"content": string(data)} } return map[string]string{"error": "data not found"} } func Responder(w http.ResponseWriter, req *http.Request) { name := req.FormValue("id") data, _ := json.Marshal(getContent(name)) w.Write(data) } func main() { http.HandleFunc("/", Responder) http.ListenAndServe(":8081", nil) } --- # Unscientific benchmark httperf, 100 clients, 1000 requests each - Python: 1900 req/s, 11 MB - PyPy: 3600 req/s, 70 MB - Go: 6100 req/s, 4 MB --- # Imports import ( "fmt" "os" "github.com/droundy/goopt" ) Supports Github, Bitbucket, Google Code, Launchpad --- # "go" tool - go build - go install - go get - go fmt - etc --- # Cross-compilation .shell $ GOOS=windows GOARCH=amd64 go build $PKG --- # Problems - Leaks on 32-bit systems - GC far from being perfect - Hard to search for - Use 'go lang' as search query --- # Questions? .center 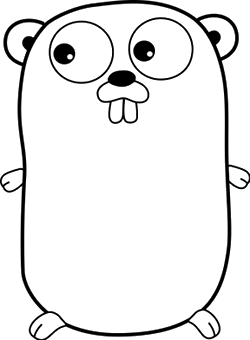 [http://golang.org/](http://golang.org/)